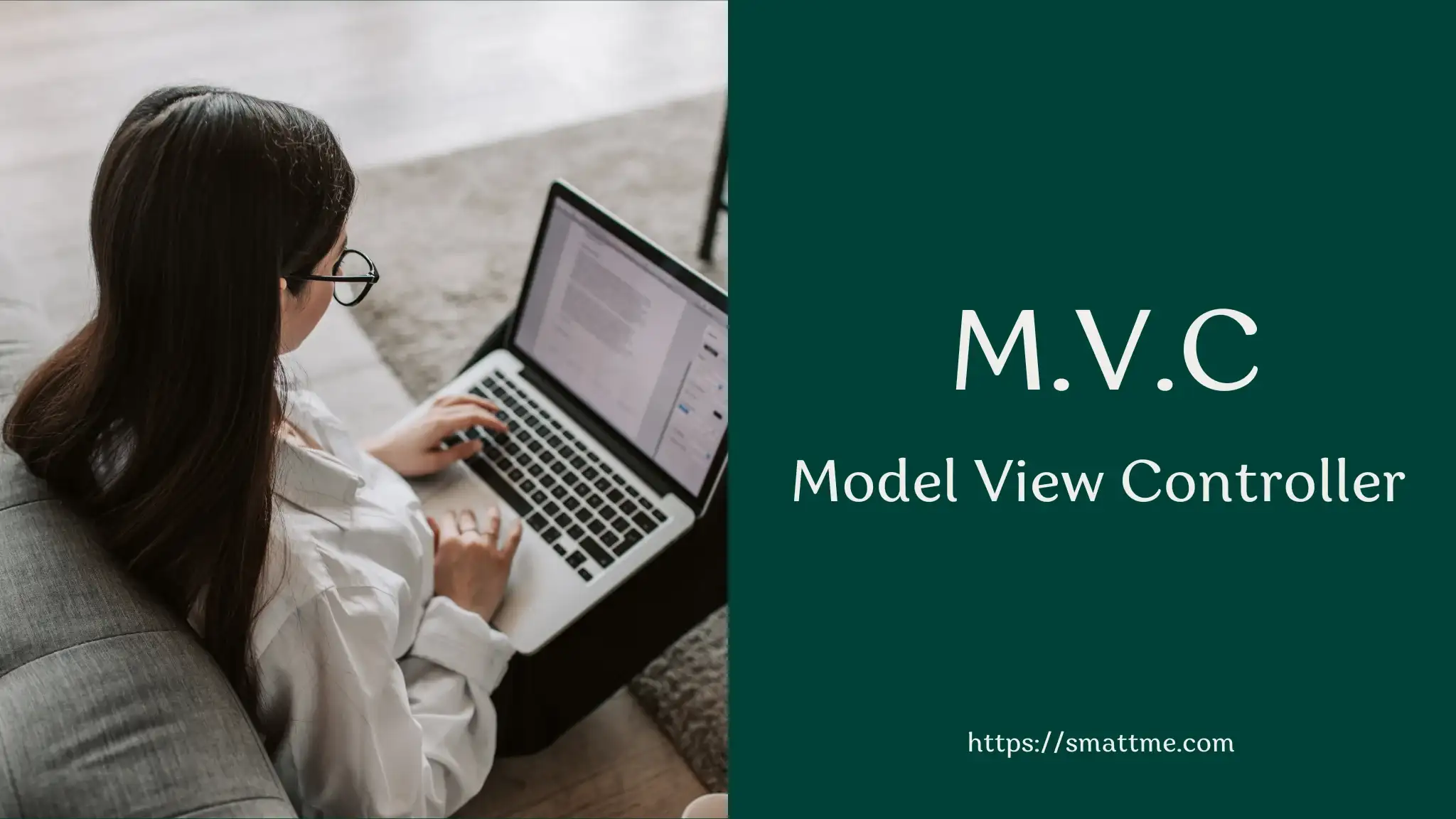
Introduction to MVC in Web Development
1. Overview
MVC (Model-View-Controller) is a design pattern that separates the major components of a web application into three distinct layers: Model, View and the Controller.
This architecture simplifies the codebase and makes it easy for different Software Engineering roles to work on the same codebase simultaneously.
In this article, we will consider each component, the role they play with examples in common web application frameworks.
2. Model
The Model is a class the represents a single row of a database table. The properties of the Model correspond to the columns of the underlying database table.
For example, a users
table with columns first_name
, last_name
and email
will have
a corresponding model class:
Listing 2.1 User.java
|
|
Note that the name of the Model is singular User
and not Users
. This is because a Model is only representing a single record of a database table.
The purpose of a Model is to make database interactions fluent and natural.
Instead of creating and using strings INSERT INTO users ...
everytime we want to
save a new users
record, we will simply instantiate a new User
Model object and then save it to the database.
The web application framework is then responsible for converting the Model into a valid SQL INSERT
statement and execute it in the database.
This is why a single web application framework like SpringBoot, Laravel, Ruby on Rails can support different databases.
Since the Engineer is not hard-coding the SQL statements, changing the database from MySQL to PostgreSQL is a matter of configuration update.
The framework will still generate the correct SQL statements from the same Models.
Another advantage of having the Model is that we can pass it as method arguments, thereby re-using the same object as opposed to creating new SQL statements everytime we need data from the database.
A Model can also refer to a class representation of an HTTP request/response, an event or a Data Transfer Object (DTO).
Another name for a Model that represents a database record is Entity as in “database Entity”.
3. View
The View - just as the name implies, is the visible component of a web application. It is the “appealing” part of a web application that the customers (or users) interact with and appreciate.
It is made up of images, texts, colours, animations, videos and even sounds in some cases.
The role of the View is pretty straightforward - to show the user/customer the end result of computations and data manipulations done at the Controller and the Model layer.
In some applications, it is the most important component, because if gotten wrong, no one will use the web app. If no one uses a web app then the business is out of business and jobs are lost.
The basic technology for creating views are HTML (Hypertext Markup Language), CSS (Cascading Style Sheet) and JavaScript. Frameworks and tools like React, Angular, SaaS, SCSS, jQuery are all built on top of the three basic technologies.
This is why HTML, CSS, and JavaScript are still basic requirements for web development. Software Engineers that specialises in working with view-related technologies are called Front-end Engineers.
Some views are static, that is, their content does not change once published e.g. https://smattme.com
. On other side of static is dynamic.
Dynamic views uses data returned from the Controller to determine what to show to the user.
The view you get when you logged in to your Gmail account is dynamic. Once you read an email, it updates the count of unread emails, among other changes.
In the context of MVC, we’re mostly concerned about dynamic views because static views do not have any Controller or Model to back them.
4. Controller
The controller is the entry point to a web application and its infrastructure. It is the component that define the URLs (Uniform Resource Locator) leading to a feature of a web application.
If you visit a URL like https://www.netflix.com/ng/login
, the /ng/login
path is defined by a Controller.
Because the Controller is among the first component to receive an HTTP request, it is where request validations take place. Request validation is the process of determining if the required parameters are part of an incoming HTTP request.
The Controller is not meant to execute business logic other than request validations. It should delegate that aspect of request processing to suitable Service classes.
Service classes are the components that contains and execute business logic.
Service class will sometimes employ the work of a ServiceHelper class and delegate some business logic execution to them. Service class will also make use of Models, Repositories, DTOs to achieve fulfil their role.
Given a social media app with the following requirements for every newly registered user:
- We need to ensure the email they want to use has not been used before by someone else
- We need to generate a referral code for their profile
- We need to check if they provide a valid referral code themselves and then reward their referrer
- We need to add their email to our CRM tool for sending them welcome email and tutorials
- and so on…
Let’s see how all the components will interact together to make it happen.
For a user to register, they will visit our website URL https://smattme.com/signup
and fill a form.
Once they submit the form, an HTTP request will be sent to POST https://smattme.com/signup
.
This URL is defined by the AuthenticationController
class.
The AuthenticationController
will receive and validate the form data, constructs a SignupRequest
object and then pass it down
to a UserService
class to register a new User.
The UserService
will execute the aforementioned business logics and at the end create a new instance of the User
Model
and save it in the database.
Furthermore, the UserService
will construct and return a suitable SignupResponse
object
to the AuthenticationController
.
The AuthenticationController
will now show the User a login view for them to log in to the app following their
successful registration.
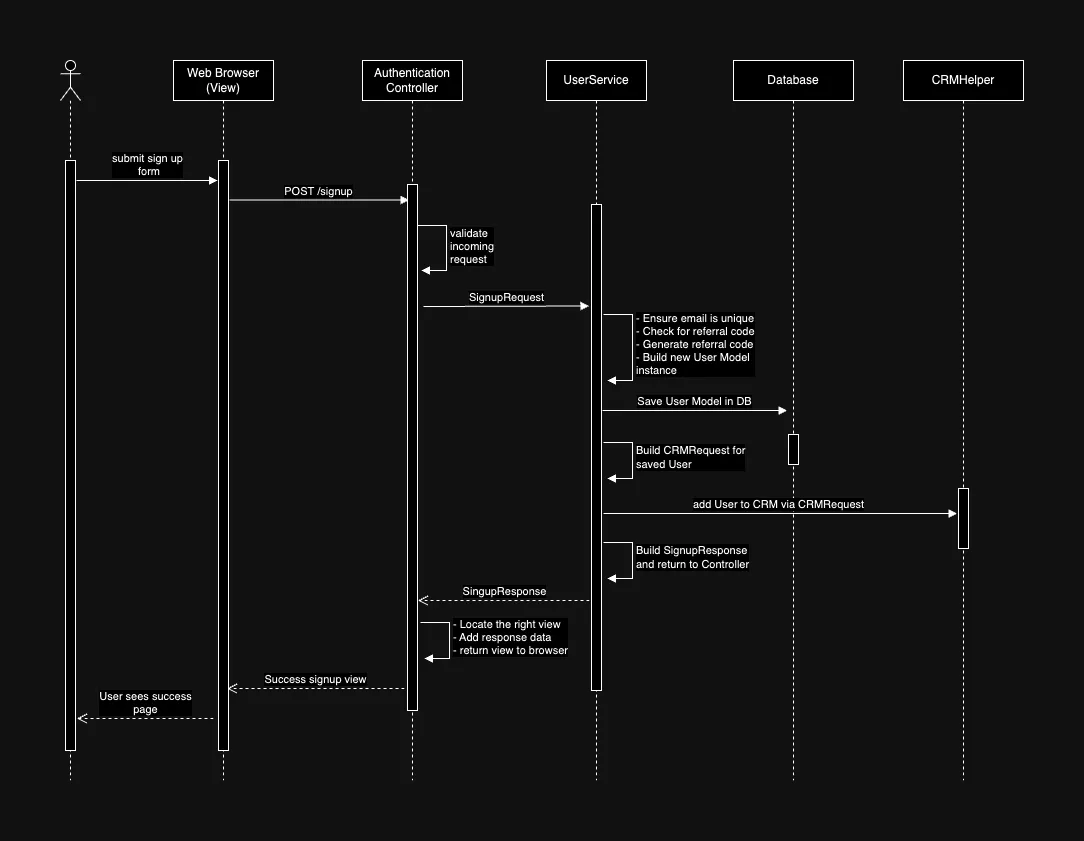
If you’ve had to sign up for any new account on the Internet, you would have experienced a flow similar to the one we described above.
In my experience, in the world of MVC, the C
- Controller, extends beyond the Controller
class itself.
It also includes the Service classes and other components that executes the business logic.
5. Conclusion
Before the advent of MVC, when everything used to be muddled together (wink wink PHP), what you find is one giant file contain several lines of codes that you dare not change one comma unless you are the author.
MVC brought some sanity into web application development by clearly separating the different layers thus making it easy to build a maintainable codebase.
There are other derivative design pattern of the MVC used in mobile and desktop application developments. Getting a grip of MVC will make understanding these other patterns easier.
Happy Coding