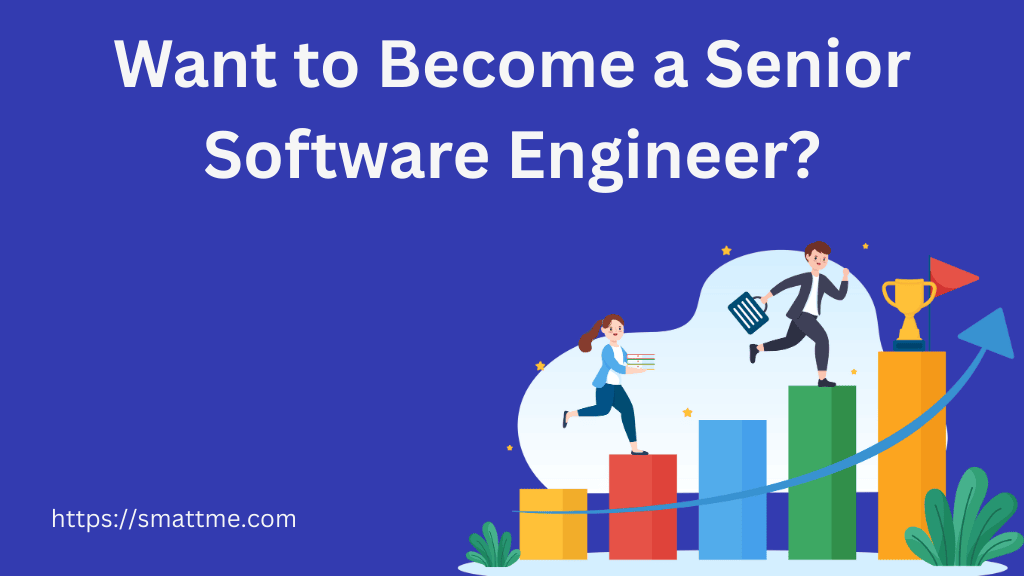
Want to Become a Senior Software Engineer? Do These Things
1. Overview
In my experience working with and leading Software Engineers, I have seen mid-level Engineers produce outcomes worthy of a Senior and Seniors that are only so in title. High-performing mid-levels eventually overtook under-performing Seniors.
How you become a Senior Software Engineer is important. If you become a Senior because you’re the last man standing or the one with the longest tenure.
I am afraid that future upward movement may be challenging. Especially, if you decide to go elsewhere.
I have been opportune to directly mentor a couple of Engineers to become Senior, and witness the journey of others.
In this article, I am going to discuss the day-to-day activities that distinguish the best, and how you can join their ranks.
2. Know the Basics
Bruce Lee famously said, “I fear not the man who has practised 10,000 kicks once, but I fear the man who has practised one kick 10,000 times.”
This is a homage to the importance of getting the basics right.
2.1 Write Clean Code
If you want to become a Senior Software Engineer, you have to write clean and reliable code.
The pull request you authored should not be like a Twitter thread due to the myriad of corrections.
Your code contributions should completely address the assigned task.
If the task is to create a function that sums two digits. In addition to the +
operation, add validations.
Take care of null
cases, and use the correct data type in the function parameters.
Think about number overflow and other cases.
This is what it means to have your code contribution address the task at hand completely.
Pay attention to the coding standard and ensure your code changes adhere to it.
When you create pull requests that do not require too many corrections, work as expected and more. You’ll be able to complete more tasks per sprint, and become one of the top contributors in the team.
You see where this is going already.
You should pay attention to the smallest details in your code. Perform null checks, and use the appropriate data types and more.
For example, in Java, do not use Integer
everywhere just because you can; it takes more memory and
may impair the performance of your application in production.
Instead of writing multiple, nested if...else
constructs, use early return.
Don’t do this:
|
|
Do this instead. It’s cleaner and more readable:
|
|
Ensure you handle different scenarios in your logic. If you are making external HTTP calls, ensure there’s exception handling that caters for 5XX and 4XX.
Validate that the return payload has the expected data points. Implement retry logic where applicable.
Write the simplest, and most performant version of your logic.
Needlessly fanciful and complicated code only impressed one person, your current self. Your future self will wonder what on earth you had to drink the day you wrote that code.
Say less about how other people will perceive it down the line.
What typically happens to such complicated code, which is not maintainable, is that it gets rewritten and deprecated.
So, if your goal is to leave a legacy behind, needlessly complicated, non-performant, hard-to-maintain code will not help.
If you’re using reactive Java programming, please do not write deeply nested code - the callback hell of JavaScript. Use functional programming to separate the different aspects and have a single clean pipeline.
2.2 Write Readable Code
In addition to writing clean code, your code should be readable.
Don’t write code as if you’re a minifier of some sort. Use white-space properly. Coding is akin to creating art. Write beautiful code that others want to read.
Use the right variable names. var a = 1 + 2;
might make sense now, until you need to troubleshoot and then
began to wonder what on earth is a
.
Now, you have to run the application in debug mode and observe the values to decode what it means.
This extra step (read extra minutes or hours) could have been avoided from the outset.
Write meaningful comments and Javadoc. Please don’t do this and call it a Javadoc:
|
|
We will be able to tell you’re the author of the code when we do Git Blame. Therefore, kindly stop adding a Javadoc to a method or class just to put your name there.
You’re contributing to the company’s codebase and not an open-source repo on GitHub. Moreover, if your contribution is substantial enough, we will definitely remember you wrote it.
Writing meaningful comments and Javadoc is all the more necessary when you’re writing special business logic.
Your comment or Javadoc can be the saving grace for your future self or colleague when that business logic needs to be improved.
I once spent about 2 weeks trying to understand the logic for generating “identifiers”. It wasn’t funny. Brilliant logic, but it took me weeks to appreciate it.
A well-written Javadoc and documentation could have saved me some hours.
Avoid spelling mistakes in variable names, comments, function names, etc. Unless your codebase is not in English, please use comprehensible English variable names.
We should not need Alan Turing to infer the function of a variable, a method, or a class from its name. Think about it, this is why the Java ecosystem seems to have long method names.
We would rather have long names with explicit meaning than require a codex to translate one.
3. Deepen your Knowledge
Software Engineering is a scientific and knowledge-based profession. What you know counts a lot towards your growth. What you know how to do is the currency of the trade.
If you want to become a Senior Software Engineer, you need to know how to use the tools and platforms employed in your organisation.
I have interviewed great candidates who did not get the maximum available offer because they only know as far as committing to the production branch.
When it comes to how the deployment pipeline works, how the logs, alerts and other observability component works, they don’t know. “That one is handled by the DevOps team”.
As a Senior Software Engineer, you need to be able to follow your code everywhere. From the product requirement, technical specification, slicing, refinement, writing, code reviews, deployment, monitoring and support. This is when you establish your knowledge and become a “Senior”.
Your organisation uses Kibana or Grafana for log visualisation, NewRelic, Datadog, etc. Do you know how to filter for all the logs for a single service? Do you know how to view the logs for a single HTTP request?
Let’s say you have an APM platform like Datadog, NewRelic or Grafana, do you know how to set up alerts?
Can you interpret an alert, or do you believe your work is limited to writing code and merging to master? While every other thing should be handled by “other people”.
If you want to become a Senior Software Engineer, you have to learn how these things are set up, how they work, be able to fix them if they break, and improve them too.
Currently, you’re not a Senior Software Engineer, but have you ever wondered what your “Senior Engineer” or “Tech Lead” had to do before assigning a task to you?
There are important steps that happen before and after the writing of the code. It is expected that a Senior Software Engineer should know them, and be able to do them well.
If your company writes technical specifications, observe refinement sessions, poker planning, or ticket slicing. Don’t be satisfied just being in attendance.
Attach yourself to someone who’s already leading these and ask to help them out. When given the opportunity, pour your heart into it. Get feedback, and you become better over time.
If you want to become a Senior Software Engineer, be the embodiment of your organisation’s coding standard. If there’s none – jackpot! Research and implement one.
In this process, you’ll move from someone who ONLY executes to someone who’s involve in the execution planning and thus ready for more responsibility, a.k.a. the Senior Software Engineer role.
Still on deepening your knowledge, you should know how the application in your custody works. One rule of thumb I have for myself is this: “Seun, if you leave this place today and someone asks you to come and build similar things, will you be able to do it?”.
It’s a simple concept but powerful. If your team has special implementations and logic somewhere that’s hard to understand, make it your job to understand them. Be the guy who knows the hard stuff.
There’s a special authentication mechanism, be the one who knows all about it. There’s a special user flow that gets confusing, be the one who knows about it off-hand.
Be the guy in Engineering who knows how the CI/CD pipeline works and is able to fix issues.
Ask yourself this: Do you know how your organisation’s deployment pipeline work, or do you just write your code and pass it on to someone else to deploy?
Without deepening your knowledge, you will not be equipped to take on more responsibilities, help during the time of an incident, or proffer solutions to major pain points.
Be the resident expert, and you can be guaranteed that your ascension will be swift.
4. Be Proactive and Responsible
I have once interviewed someone who seems to have a good grasp of the basics and the coding aspect. However, we were able to infer from their submissions that they’ve never led any project before.
While they may be a good executor, they’re not yet ready for the Senior role.
Volunteer and actively seek opportunities to lead initiatives and do hard things in your organisation. Your team is about to start a new project, or build a new feature? Volunteer to be the technical owner.
When you are given the opportunity, give it your best. Write the best technical specification there is, and review pull requests from other people in time.
Submit the most code contributions, organise and carry out water-tight user acceptance tests.
When the feature/project is in production, follow up with it to ensure it is doing exactly what it is supposed to do, and delivering value for the business.
Do these, and now you have things to reference when your name comes up for a promotion.
Furthermore, take responsibility for team and organisational challenges.
For example, no one wants to update a certain codebase because the test cases are flaky and boring. Be the guy who fixes that without being asked.
Of course, solving a problem of that magnitude shows you as a dependable team member, who is hungry for more.
Another example, your CI/CD pipeline takes 60 minutes to run. Why not be the person who takes some time out to optimise it.
If you get it from 60 minutes to 45 minutes, that’s a 25% improvement in the pipeline. If we compute the number of times the job has to run per day, and multiply that by 5 days a week.
We are looking at saving 375 minutes of man-hours per day. Holy karamba! That’s on a single initiative. Now, that’s a Senior-worthy outcome.
I’m sure, if you look at your Engineering organisation, there are countless issues to fix and things to improve. You just need to do them.
Another practical thing you can do to demonstrate proactivity and responsibility is simply being available.
You most likely have heard something about “the available becomes the desire”.
There’s an ongoing production incident, and a bridge call. Join the call. Try to contribute as much as possible to help solve the problem.
Attend the post-incident review calls and contribute. You see, by joining these calls, you’ll see and learn how the current Seniors troubleshoot issues.
You’ll see how they use the tools and you’ll learn a thing or two.
It may not be production incident, it may be a customer support ticket, or an alert on Slack about something going wrong.
Don’t just “look and pass” or suddenly go offline. Show some care, attempt to fix it, you can only get better at it.
The best thing about getting better at it is that you become a critical asset to the company. While it is true that anyone is expendable, provided the company is ready to bear the cost.
I have also been in a salary review session where some people get a little bit more than others, on the same level, because they’re considered a “critical asset”.
It’s a thing, and either you know it or not, it applies.
Be proactive (do things without being told to do so) and be responsible (take ownership), and see yourself grow beyond your own imaginations.
5. Be a Great Communicator
Being a great communicator is crucial to your career progression to the Senior Software Engineer role. The reason is that, you work with other human beings, and they are not mind readers.
Are you responding to the customer support ticket or responding to an alert on Slack? Say so in that Slack thread so other people can do something else.
Are you blocked? Please say so. Mention what exactly you have tried and ask for ideas.
We don’t want to find out on the eve of the delivery date that you’ve been stuck for the past 1 week, and so the team won’t be able to meet their deadline.
When other people ask for help, and you are able to, please unblock them. You only get better by sharing in your team.
Adopt open communication as much as possible. It will save you from having to private message 10 different people, on the same subject, to reach a decision.
Just start a Slack thread in the channel, and everyone can contribute and reach a decision faster.
It also helps with accountability and responsibility.
6. What If?
Seun Matt, “What if I do all these things and still do not get promoted? I am the resident expert, I know my stuff, I am proactive and a great communicator. I have the basics covered, and I even set the standards. Despite all of this, I have not been promoted in years.”
I hear you loud and clear, my friend. We have all been there at some point.
There are times when an organisation is not able to perform pay raises or do promotions due to economic challenges, lack of profitability and other prevailing market forces.
Remember, the companies we work for do not print money, it is from their profit that they do promotions, raises and bonuses.
For you, it is a win-win situation no matter how you look at it. These skill sets that you now have. The path you have taken, they are all yours and applicable in the next company.
In this profession, your next Salary is influenced by what you’re doing at your current place and what you’ve done in the past.
So, even if it does not work out in your current place, when you put yourself out there, you’ll get a better offer—all things being equal.
5. Conclusion
No matter where you work, ensure you have a good experience. “I have done it before” trumps the number of years.
In Software Engineering, experience is not just about the number of years you’ve worked in a company or have been coding.
Experience has to do with the number of challenges you have solved yourself. How many “I have seen this before” you have under your belt?
Being passive or just clocking in 9 - 5 will not get you that level of experience. You need to participate and lead.
The interesting part is that, your next Salary in your next job will be determined by the experience you’re garnering in your current work.
Doing all of the above is a call of duty. It requires extra work and it has extra rewards for those able to pursue it.
Stay curious, see you at the top and Happy Coding!