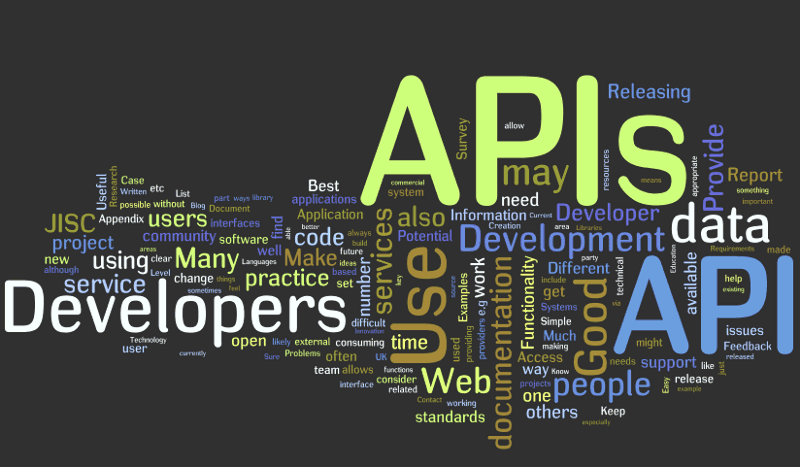
Formstack API Tutorial: Working with fstackapi_php library
Overview
In this article, we will be looking at how to work with the Formstack’s REST API v2. We will be using the fstackapi_php PHP package that I built to simplify the process.
How does Formstack’s API Work?
Formstack is an online form building platform. Formstack API is organised around objects that are involved in form creation and submission.
A user logs into the platform, create a Form that has Fields for collecting data and share the link to the form with the target audience that can access the Form, fill in their data and make a final submission.
How to programmatically Submit a Form to Formstack
Visit fstackapi_php repo on GitHub and follow the instructions to install and configure the package
Get the id of the form you want data to be submitted to. We can do this by first getting all the forms that are available to our account and taking note of the particular form id we are interested in. It is advisable to save the returned values in a file for reference’s sake:
|
|
Then, let’s get the id of the fields that are associated with the form. The way Formstack works is that each Form
has associated Fields
;
and each Field
is an object with id
, name
, label
and other attributes.
We have the formId
from the snippet above. So let’s get the Fields
for the form:
|
|
It is also advisable that we save the returned ids for use later.
Now that we have the formId
, and id
of the fields. Let’s construct a data and submit it to the Form
via the library.
Let’s assume our form has only two fields: name
(id
= 123433
) and email
(id
= 4312232
).
Note here, that the format for specifying the field is field_$fieldId
. Where $fieldId
is the id
of a particular field.
If the prefix field_
is omitted, the form submission will not reflect in Formstack’s database:
|
|
And that’s it — very simple, right? Yeah! The fstackapi_php library makes the process seamless. What if we want to get all the submissions? Well, we only need to call this method:
|
|
and this method will get the detail of a single submission:
|
|
There are other methods and classes that enable you to work with folders, fields, forms and submissions. They are well documented in the GitHub repo of fstackapi_php, remember to give it a star.
There is a chance you may not be working with Formstack API as at present. Feel free to recommend this to others, in your network who might need them now.
Happy Coding